Learn Python Programming An Advanced Journey Plus 72 Exercises
04
March
2025
4.38 GB | 14min 33s | mp4 | 1280X720 | 16:9
Genre:eLearning |Language:English
Files Included :
1 -Introduction.mp4 (25.59 MB)
10 -Coding exercise #9 multiple exceptions, index error.mp4 (51.87 MB)
11 -Coding exercise #10 Bank simulation system for withdrawal.mp4 (52.53 MB)
12 -Coding exercise #11 Login system simulation.mp4 (71.63 MB)
2 -Coding exercise #1 Try-except block.mp4 (38.34 MB)
3 -Coding exercise #2 Catching specific exceptions.mp4 (59.44 MB)
4 -Coding exercise #3 Multiple exceptions.mp4 (41.52 MB)
5 -Coding exercise #4 Finally blocks.mp4 (58.17 MB)
6 -Coding exercise #5 Else blocks.mp4 (52.23 MB)
7 -Coding exercise #6 Custom exception.mp4 (50.36 MB)
8 -Coding exercise #7 Catching division by zero.mp4 (24.36 MB)
9 -Coding exercise #8 Invalid input.mp4 (41.04 MB)
1 -Introduction to classes.mp4 (67.42 MB)
10 -Coding exercise #20 Managing student record system.mp4 (71.82 MB)
11 -Coding exercise #21 Library system.mp4 (55.41 MB)
12 -Coding exercise #22 Car class.mp4 (52.53 MB)
13 -Coding exercise #23 Class attributes.mp4 (47.26 MB)
14 -Coding exericse #24 Class method.mp4 (51.6 MB)
15 -Introduction to encapsulation.mp4 (52.16 MB)
16 -Coding exercise #25 Public attributes.mp4 (23.24 MB)
17 -Coding exercise #26 Protected attributes.mp4 (61.38 MB)
18 -Coding exercise #27 Private attributes.mp4 (106.38 MB)
19 -Coding exercise #28 Getter and setter method.mp4 (90.76 MB)
2 -Coding exercise #12 Priting different messages using a class.mp4 (38.45 MB)
20 -Coding exercise #29 Encapsulation for Tesla.mp4 (155.1 MB)
3 -Coding exercise #13 Calculation of area for different shapes.mp4 (62.1 MB)
4 -Coding exercise #14 Convertor class.mp4 (63.69 MB)
5 -Coding exercise #15 Test processor class.mp4 (91.77 MB)
6 -Coding exercise #16 Constructors and destructors.mp4 (162.12 MB)
7 -Coding exercise #17 Constructors and destructors 2.mp4 (75.92 MB)
8 -Coding exercise #18 Bank account system.mp4 (59.65 MB)
9 -Coding exercise #19 Shopping cart.mp4 (80.02 MB)
1 -Introduction.mp4 (15.3 MB)
2 -Coding exercise #30 Single inheritance.mp4 (44.23 MB)
3 -Coding exercise #31 Multiple inheritance.mp4 (28.42 MB)
4 -Coding exercise #32 Multi-level inheritance.mp4 (32.53 MB)
5 -Coding exercise #33 Hierarchical inheritance.mp4 (27.92 MB)
6 -Coding exercise #34 Hybrid inheritance.mp4 (50.42 MB)
7 -Coding exercise #35 Super() method.mp4 (37.03 MB)
8 -Coding exercise #36 Method resolution order.mp4 (71.12 MB)
9 -Coding exercise #37 Default arguments.mp4 (35.6 MB)
1 -Introduction to polymorphism.mp4 (15.88 MB)
2 -Coding exercise #38 Overriding.mp4 (49.37 MB)
3 -Coding exercise #39 Overloading a shape class.mp4 (49.47 MB)
4 -Coding exercise #40 Overloading a text message.mp4 (62.55 MB)
5 -Coding exercise #41 Overloaded add.mp4 (62.22 MB)
6 -Coding exercise #42 Some overloadded functions.mp4 (52.4 MB)
7 -Coding exercise #43 Polymorphism with functions and objects.mp4 (87.84 MB)
8 -Coding exercise #44 Duck typing.mp4 (32.85 MB)
1 -Collection module.mp4 (78.35 MB)
10 -Coding exercise #53 Namedtuple for storing employees' data.mp4 (34.63 MB)
11 -Coding exercise #54 Namedtuple for color format.mp4 (41.12 MB)
12 -Coding exercise #55 Namedtuple and functions.mp4 (49.24 MB)
13 -Coding exercise #56 Converting a Named tuple.mp4 (41.01 MB)
14 -Coding exercise #57 Using the replace() function for namedtuples.mp4 (39.92 MB)
15 -Coding exercise #58 Working with deques in Python.mp4 (120.17 MB)
16 -Coding exercise #59 First-in-First-Out in deques.mp4 (20.17 MB)
17 -Coding exercise #60 Last-in-First-Out in deques.mp4 (14.48 MB)
18 -Introduction to `ordered dictionaries'.mp4 (7.17 MB)
19 -Coding exercise #61 Everything about ordered dictionaries.mp4 (159.26 MB)
2 -Coding exercise #45 Counter for different strings.mp4 (52.6 MB)
20 -Coding exercise #62 Creating and accessing elements of an ordered dictionary.mp4 (20.06 MB)
21 -Coding exercise #63 Task completion system.mp4 (21.59 MB)
22 -Introduction to chainmaps.mp4 (8.14 MB)
23 -Coding exercise #64 Everything about chainmaps.mp4 (149.68 MB)
24 -Coding exercise #65 Managing configuration settings.mp4 (54.51 MB)
25 -Coding exercise #66 Variable scope resolution.mp4 (52.1 MB)
26 -Introduction to heapq.mp4 (7.53 MB)
27 -Coding exercise #67 min heap.mp4 (17.3 MB)
28 -Coding exercise #68 Adding and removing elements from a heap.mp4 (50.27 MB)
29 -Coding exercise #69 Prority queue using heaps.mp4 (38.02 MB)
3 -Coding exercise #46 Counting words and letters.mp4 (84.5 MB)
30 -Coding exercise #70 Finding the smallest element.mp4 (20.55 MB)
31 -Coding exercise #71 Finding the largest element.mp4 (15.17 MB)
32 -Coding exercise #72 How min heap works.mp4 (27.69 MB)
4 -Coding exercise #47 Defaultdict(int).mp4 (63.25 MB)
5 -Coding exercise #48 Defaultdict(list).mp4 (125.15 MB)
6 -Coding exercise #49 Defaultdict(set).mp4 (103.79 MB)
7 -Coding exercise #50 Namedtuple.mp4 (66.5 MB)
8 -Coding exercise #51 Namedtuple for GPS coordinates.mp4 (55.76 MB)
9 -Coding exercise #52 Namedtuple for student record system.mp4 (51.27 MB)]
Screenshot
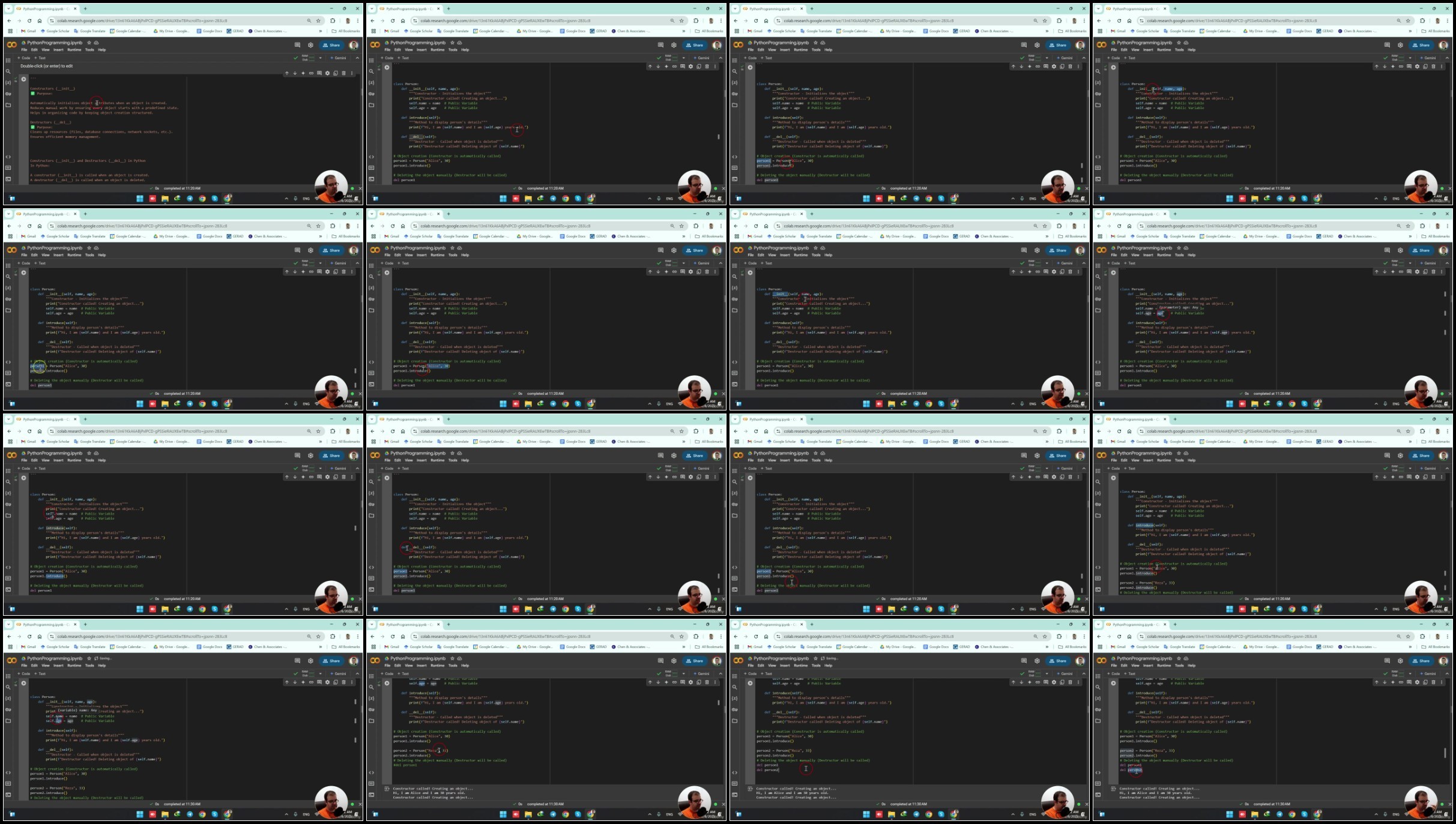
RapidGator
https://rapidgator.net/file/1d30e1987ccc14c2e5254e5eb9c6faa7/
https://rapidgator.net/file/4287a76a471c6e824fdbb33a557b1c62/
https://rapidgator.net/file/5d68fdeeec065b272db89893c9ce45e3/
https://rapidgator.net/file/14b5698f6cf5f423e5156bb5653d839e/
https://rapidgator.net/file/5e4ba4872fd03d4005e5072911865560/
NitroFlare
https://nitroflare.com/view/C3F3F0A2D1C6BE7/
https://nitroflare.com/view/F3DA064CCBBEE71/
https://nitroflare.com/view/1B3D1E3159D17A9/
https://nitroflare.com/view/14E8E056FD90F35/
https://nitroflare.com/view/E93A08AD890B054/
Note:
Only Registed user can add comment, view hidden links and more, please register now
Only Registed user can add comment, view hidden links and more, please register now
Related Posts